Hosted Payment Page
You’re just a few steps away from integrating our Hosted Payment Page (HPP), offering your customers an intuitive, seamless checkout experience.
Why will you love this solution?
Secure
Now, being secure is that much easier. With our HPP, there’s only PCI DSS SAQ A (that’s Payment Card Industry Data Security Standard Self-Assessment Questionnaire A, in case you wondered) to complete. You get the customers; we have your security.
Dynamic
There’s no need to reinvent the design wheel. Our dynamic templates adapt to your display requirements for device and screen size.
Personal
Make your payment page as handsome as the rest of your site. Upload HTML, CSS, JavaScript, image, and font files to our secure environment and watch magic happen.
Let's get started.
Configuration
Your unique HPP gets created in the Merchant section of our portal. To build or upload an HPP for a specific merchant ID (MID), go to Merchant > Company & MID > Create Service. Not sure if you’re in the right place? Take a look at the screenshot below:
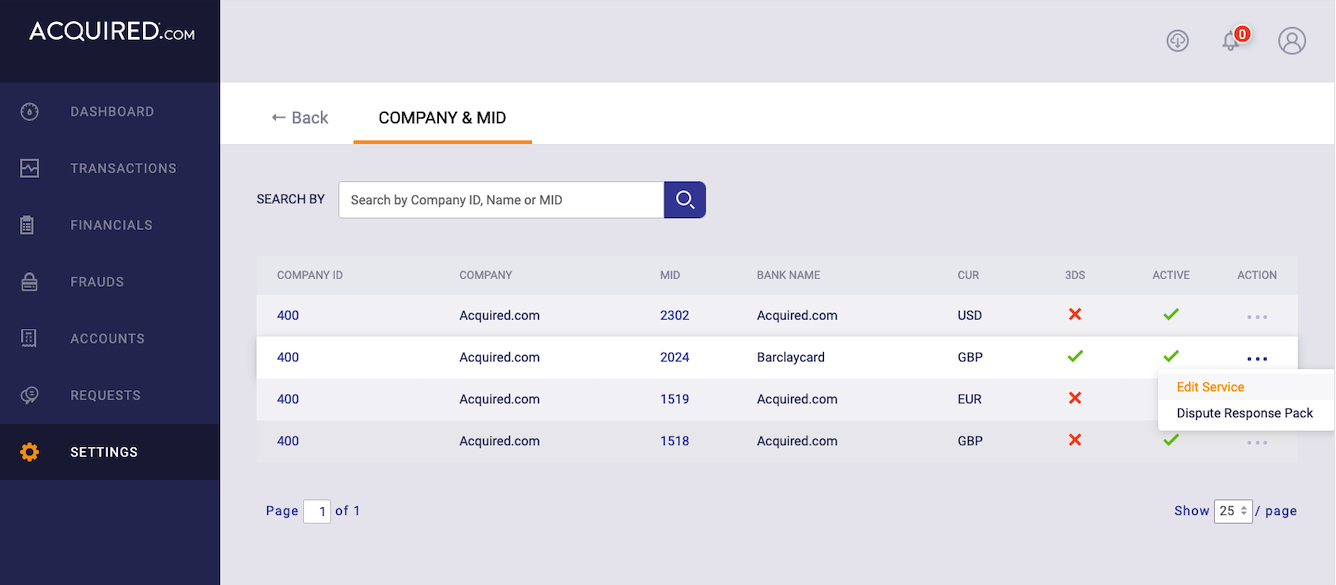
Simple as that. Now it’s time to customise your payment page. We’ve also added a default template to the dashboard to get you started. Download it and if you need some help, take a look here.
Endpoints
Time to play - access your HPP using these endpoints:
Security
For your sake and ours, we make sure that each transaction you send to us is a genuine request.
Here is how:
Verified Referring URL
Your customers are redirected to the HPP using a referring URL. We only accept requests from referring URLs whitelisted within the Hub, so make sure you go to Merchants > Company & MID > HPP > Edit and do so, so that we know you’re for real.
Request Hash
We use encrypted tokens to assess the integrity of your transaction data. This encrypted token is referred to as the Request Hash and sent in the hash
parameter of your request to us. If data does not pass our integrity check because the hash value is missing or mismatches the transaction data, we decline the transaction request. It’s not personal, it’s just part of the process of keeping everything as secure as acceptable. Each transaction request needs a unique hash value, so here are the steps to start generating yours:
-
Sort the transaction parameters - excluding your company hash - alphabetically and then concatenate (don’t you just love this word?). Here are some examples:
amount . company_id . company_mid_id . currency_code_iso3 . merchant_order_id . transaction_type //14.991131045GBP20170619111AUTH_CAPTURE
Over here at Request Parameters, we’ve listed all the parameters to include with each transaction request.
-
SHA256 encrypt the resulting string:
24febfefcd5fd890837e82b8eb29759227f7614f4bc99ce0ea9af63399cfacad
-
Then, append your company_hashcode to the string generated in step two and SHA256 encrypt again:
24febfefcd5fd890837e82b8eb29759227f7614f4bc99ce0ea9af63399cfacadcompany_hashcode
7c93225890c7cbdf9eb904285c18fa9b72d47acd3e007049fb9adbad1fe59f27
Pro tip: It’s time to get your company_hashcode (if you haven't already). Send the support@acquired.com team a note, they’ll allocate one straight away.Please note: When processing via the HPP you have to include all the parameters included in the request when calculating yourhash
value.
With us so far? To get you started, here are samples of code to generate encrypted hash values:
?php
$param = array(
'company_id'=>133,
'company_mid_id'=>1045,
'merchant_order_id'=>'20170619111',
'transaction_type'=>'AUTH_CAPTURE',
'currency_code_iso3'=>'GBP',
'amount'=>14.99,
);
$company_hashcode ='company_hashcode';
ksort($param);
$plain = implode('', $param);
// $plain ="14.991131045GBP20170619111AUTH_CAPTURE";
$temp = hash('sha256', $plain);
// $temp = "0722b76a63d0b7adde39b13119e4dfd5aa7bd0d91885d55597af334a055c4cd0";
$hash = hash('sha256', $temp.$company_hashcode);
//$hash = "2934149504e39101f5cfad6ec3e971978890a057373ac3237e0dd150dd491d3d3"
?>
private String getHppHash() {
String companyHash="hashcode";
String hppHash="";
Map map = new HashMap();
map.put("amount", 100.00);
map.put("companyId", 133;
map.put("companyMidId", 1025);
map.put("currencyCodeIso3", "GBP");
map.put("merchantOrderId", "20160907_111");
map.put("transactionType", "AUTH_ONLY");
Map sortMap = new TreeMap(new MapKeyComparator());
sortMap.putAll(map);
StringBuffer sb = new StringBuffer();
for (Iterator iterator = sortMap.entrySet().iterator(); iterator.hasNext(); ) {
Map.Entry entry = (java.util.Map.Entry) iterator.next();
sb.append(null == entry.getValue() ? "" :
entry.getValue().toString());
}
String plain = sb.toString();
// plain = "1001331025GBP20160709_666AUTH_ONLY";
String temp = String2SHA256(sb.toString());
// temp = "137fe6414788bbe12676555889ae3688edc5d2819394a3e284a1b022bb2648cb";
hppHash = String2SHA256( temp + companyHash);
// hppHash = "2fa91f0d42ff36c18c089d27dba4201be9b670073b0b95dd822e7c3038aeeaf5";
}
private static String String2SHA256(String str) {
MessageDigest messageDigest;
String encodeStr = "";
try {
messageDigest = MessageDigest.getInstance("SHA-256");
messageDigest.update(str.getBytes("UTF-8"));
encodeStr = byte2Hex(messageDigest.digest());
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
return encodeStr;
}
private static String byte2Hex(byte[] bytes) {
StringBuffer stringBuffer = new StringBuffer();
String temp = null;
for (int i = 0; i < bytes.length; i++) {
temp = Integer.toHexString(bytes[i] & 0xFF);
if (temp.length() == 1) {
stringBuffer.append("0");
}
stringBuffer.append(temp);
}
return stringBuffer.toString();
}
class MapKeyComparator implements Comparator {
@Override
public int compare(String str1, String str2) {
return str1.compareTo(str2);
}
}
public static void Main(string[] args)
{
string companyHashCode = "hashcode";
Dictionary param = new Dictionary
{
{ "company_id", 133 },
{ "company_mid_id", 1025 },
{ "merchant_order_id", "20160709_666" },
{ "transaction_type", "AUTH_ONLY" },
{ "currency_code_iso3", "GBP" },
{ "amount", 100.00 }
};
Dictionary sorted = param.OrderBy(o => o.Key).ToDictionary(o => o.Key, p => p.Value);
string plain = string.Empty;
foreach (KeyValuePair k in sorted)
{
plain += k.Value;
}
// plain = "1001331025GBP20160709_666AUTH_ONLY";
string temp = SHA256(plain);
// temp = "137fe6414788bbe12676555889ae3688edc5d2819394a3e284a1b022bb2648cb";
string hppHash = SHA256(temp + companyHashCode);
// hppHash = "2fa91f0d42ff36c18c089d27dba4201be9b670073b0b95dd822e7c3038aeeaf5";
}
public static string SHA256(string str)
{
byte[] SHA256Data = Encoding.UTF8.GetBytes(str);
SHA256Managed Sha256 = new SHA256Managed();
byte[] by = Sha256.ComputeHash(SHA256Data);
return BitConverter.ToString(by).Replace("-", "").ToLower(); //64
}
Request
The last page of your website before customers reach our environment is the referral page. This is the place to integrate a form with hidden HTML fields containing customers’ order data. We receive the order data via POST
request. The payment page loads, and customers are prompted to enter their sensitive card data. And that’s it, just like that you’ve completed a payment!
<html>
<head>
<title>Sample Code</title>
</head>
<body>
<form action="https://qahpp.acquired.com" method="POST">
<input type=hidden name="company_id" value="113">
<input type=hidden name="company_mid_id" value="1045">
<input type=hidden name="currency_code_iso3" value="GBP">
<input type=hidden name="transaction_type" value="AUTH_CAPTURE">
<input type=hidden name="merchant_order_id" value="20170619111">
<input type=hidden name="amount" value="14.99">
<input type=hidden name="hash" value="2934149504e39101f5cfad6ec3e971978890a057373ac3237e0dd150dd491d3d3">
<input type=submit value="Submit">
</form>
</body>
</html>
Request Parameters
Every HPP transaction requires a certain number of mandatory fields, which you’ll see at the top of the table below. Minimal data will work, but we recommend that you give us extra information with each request. This fulfils additional security checks such as Account Verification System (AVS), where available.
Parameter | Format | Length | Description | |
---|---|---|---|---|
company_id Required |
int |
1-4 | API Company ID we issue to merchants. Acceptable Characters: 0-9 |
|
company_mid_id Required |
int |
1-11 | API Merchant ID we issue to merchants. Acceptable Characters: 0-9 |
|
hash Required |
string | 64 | Value of your encrypted token. Take a look here for more information. Acceptable Characters: a-z A-Z 0-9 |
|
vt | boolean |
1 | Additional parameter to flag a transaction as MOTO - include if processing payments via phone or an IVR solution. Acceptable Characters: 0/1 |
|
merchant_order_id Required |
string | 1-50 | Unique ID you’ll use to identify each transaction. Acceptable Characters: a-z A-Z 0-9 _ - |
|
transaction_type Required |
string | 1-20 | Possible values are AUTH_ONLY or AUTH_CAPTURE. Please see here for more information. Acceptable Characters: a-z A-Z _ |
Click to list all possible transaction types. |
currency_code_iso3 Required |
string | 3 | Transaction currency, an ISO 4217 3-digit code. Acceptable Characters: a-z A-Z |
|
amount Required |
int |
1-11 | Transaction amount. Acceptable Characters: 0-9 in the format DDDDDD.CC |
|
is_tds EMV 3-D Secure (v2) |
int |
1 | Flag to initiate 3-D Secure / SCA authentication. Should be set to 2 to enable both versions of 3-D Secure. Acceptable Characters: 0/2 |
|
merchant_customer_id | string | 0-50 | Unique ID you’ll use to identify each customer. Acceptable Characters: a-z A-Z 0-9 , . - _ |
|
merchant_custom_1 | string | 0-50 | You can send additional data relating to the customer or another internal reference - with more data, your internal teams will be able to identify the customer’s transactions. Acceptable Characters: a-z A-Z 0-9 , . - _ |
|
merchant_custom_2 | string | 0-50 | You can send additional data relating to the customer or another internal reference - with more data, your internal teams will be able to identify the customer’s transactions. Acceptable Characters: a-z A-Z 0-9 , . - _ |
|
merchant_custom_3 | string | 0-50 | You can send additional data relating to the customer or another internal reference - with more data, your internal teams will be able to identify the customer’s transactions. If you are using our dynamic descriptor feature, you will not be able to use the merchant_custom_3 field as the dynamic descriptor value should be passed in this field.Acceptable Characters: a-z A-Z 0-9 , . - _ |
|
customer_fname | string | 0-50 | Customer's first name. Acceptable Characters: a-z A-Z , . - ' |
|
customer_lname MCC 6012 |
string | 0-50 | Customer's last name. Acceptable Characters: a-z A-Z , . - _ |
|
customer_dob MCC 6012 |
string | 10 | Customer’s date of birth. Acceptable Characters: 0-9 in the format YYYY-MM-DD |
|
billing_street AVS & EMV 3DS (Recommended) |
string | 0-50 | Cardholder’s street address. Acceptable Characters: a-z A-Z 0-9 , . - _ / \ & |
|
billing_street2 | string | 0-50 | Cardholder’s street address, line 2. Acceptable Characters: a-z A-Z 0-9 , . - _ / \ & |
|
billing_city EMV 3DS (Recommended) | string | 0-100 | City as it appears in a cardholder’s address. Acceptable Characters: a-z A-Z 0-9 , . - _ / \ & |
|
billing_state | string | 0-3 | State or province as it appears in a cardholder’s address. Should only be sent when processing US cards. Acceptable Characters: a-z A-Z 0-9 , . - _ |
|
billing_zipcode MCC 6012 & AVS & EMV 3DS (Recommended) |
string | 0-100 | Cardholder’s billing ZIP or postal code. Acceptable Characters: a-z A-Z 0-9 , . - _ |
|
billing_country_code_iso2 EMV 3DS (Recommended) |
string | 2 | The ISO 3166 2-character country code of the cardholder’s address. Acceptable Characters: a-z A-Z |
|
billing_phone_code | string | 3 | Cardholder’s billing phone country code (For example, 44 for the UK). Acceptable Characters: 0-9 ( ) - |
|
billing_phone |
string | 7-15 | Cardholder’s billing phone number - we strongly recommend sending the customer's mobile phone number. Acceptable Characters: 0-9 ( ) - |
|
billing_email EMV 3DS (Recommended) | string | 0-254 | Cardholder’s billing email address. Acceptable Characters: + 0-9 a-z A-z _ - . @ |
|
callback_url | string | 10-2083 | For more information about direct server-to-server communication, click here. This parameter will overwrite the default value configured within the Hub. Acceptable Characters: 0-9 a-z A-z - . _ ~ : / ? # [ ] @ ! $ & ' ( ) * + , ; = ` . |
|
return_url | string | 10-2083 | URL to redirect customers from our environment back to your website after a request has been completed. This parameter will overwrite the default value configured within the Hub. Acceptable Characters: 0-9 a-z A-z - . _ ~ : / ? # [ ] @ ! $ & ' ( ) * + , ; = ` . |
|
return_method | string | 0-12 | Specify how to receive a response back to your return_url. Acceptable values are POST , GET , POST_MESSAGE . This parameter will overwrite the default value configured within the Hub. |
|
error_url | string | 10-2083 | Customers will be redirected to this URL when an error has occurred. Sent as a GET response, we return the following parameters: status, message, company_id, company_mid_id, and merchant_order_id.Acceptable Characters: 0-9 a-z A-z - . _ ~ : / ? # [ ] @ ! $ & ' ( ) * + , ; = ` . |
|
cancel_url | string | 10-2083 | Action for a ‘Cancel’ button if your payment page has one. This parameter will overwrite the default value configured within the Hub. This does not apply if integrating via i-Frame/SDK. Acceptable Characters: 0-9 a-z A-z - . _ ~ : / ? # [ ] @ ! $ & ' ( ) * + , ; = ` . |
|
load_balance | boolean |
1 | Flag to notify our platform that you want to use our Load Balancing functionality. For more information on this feature, please see here. Acceptable Characters: 0/1 |
|
template_id | int | 0-4 | You can have multiple HPP templates to cater to your customers devices i.e. Dark Mode/Light Mode. If included, this parameter will overwrite the default template configured within the Hub. Acceptable Characters: 0-9 |
|
merchant_contact_url EMV 3DS (Required) | string | 1 | A link to the Contact Us page on your website for example https://yourdomain/contact. Acceptable Characters: 0-9 a-z A-z - . _ ~ : / ? # [ ] @ ! $ & ' ( ) * + , ; = ` . |
|
tds_source EMV 3DS (Required) | int | 1 | The channel being used to initiate the transaction, should be set to 1. Acceptable Characters: 0-9 |
|
tds_type EMV 3DS (Required) | int | 1 | The type of transaction you are processing, value should be set to 2.Acceptable Characters: 0-9 | |
tds_preference EMV 3DS (Optional) | int | 1 | If you'd like the customer to be challenged: 0 = No Preference 1 = Don't Challenge 2 = Request Challenge The issuer may decide to ignore your preference. For requests where card details are being stored for future use, this value must be set to: 2 = Request Challenge. |
Authorisation
Card transactions result in one of two transaction_type
parameters for authorisation:
AUTH_ONLY
verifies the customer account and okays the transaction now. The payment completes when you process theCAPTURE
later.AUTH_CAPTURE
performs both actions in one step to process the payment now.
Error Handling
If there is something wrong with your request, or something goes wrong on our end, we'll redirect the user to the Error URL to let you know. This will be sent as a GET here is a handy example:
https://yourdomain.com/error_url?status=500&message=hash+is+invalid.&company_id=113&company_mid_id=1045&hpp_id=27802&merchant_order_id=errortesting-92380&company_name=DemoCompany&merchant_name=DemoMerchant
Response
We have cleverly enabled two ways you can receive transaction authorisation results in our hosted environment. And even more clever, these can be used in tandem, ensuring that responses are not lost due to inconvenient circumstances such as connection issues between us and your servers.
Return URL
We will redirect your customer back to your environment using the Return URL you specify after the payment has been completed. When configuring the HPP, you can set a default value in the Hub or you can dynamically change the Return URL by passing through the return_url
parameter within the request.
We will send the message to your Return URL via a POST or GET response. We do recommend POST as GET can only send through a limited number of parameters - namely:
amount, avsaddress, avszipcode, code, company_id, company_mid_id, currency_code_iso3, cvvresult, is_remember, merchant_custom_1, merchant_custom_2, merchant_custom_3, message, transaction_id and hash.
When using POST
- we will send through everything that is sent in the request and gathered on the HPP.
POST_MESSAGE
to the parent frame.
We can only send the following parameters due to security concerns but all parameters will be sent to your Callback URL.
amount, avsaddress, avszipcode, code, company_id, company_mid_id, currency_code_iso3, cvvresult, is_remember, merchant_custom_1, merchant_custom_2, merchant_custom_3, message, transaction_id and hash.
For more information as to what the response message will look like, please refer to the code block below.
{
"amount":"10",
"authorization_code":"123456",
"avsaddress":"M",
"avszipcode":"NP",
"bin":"402400",
"cardexp":"102020",
"cardnumber":"XXXX-XXXX-XXXX-8280",
"code":"1",
"company_id":"111",
"company_mid_id":"1111",
"currency_code_iso3":"GBP",
"cvvresult":"M",
"is_remember":"1",
"transaction_id":"123456",
"hash":"490789ed723ac8c311c6e8f34fcf3f26428ed47aaa17450060acea2e90a85048"
}
Callback URL
For every payment processed via the HPP, we will send a message to your Callback URL. We introduced the Callback URL for direct server-to-server communication to ensure you receive the response every single time.
Scenarios where you may not receive a response to the Return URL or a POST_MESSAGE are:
- The customer closed their browser before being redirected back to your site.
- There was a temporary connection issue with your servers.
- A general issue with internet communication.
When configuring the HPP within the Hub, you can set a default Callback URL value or you can dynamically change the endpoint for each individual request by setting the callback_url
parameter.
We must receive a HTTP 200 OK response back from your server. If we do not, we will ping you five times in five-minute intervals until we do to ensure your system is updated.
callback_url
must begin with https://
and should be configured to handle all of our possible Response Codes.
You guessed it, the callback_url
parameter must begin with https:// and it should be configured to handle all possible transaction response codes, including errors.
Verifying the Response
You will spot the parameter hash in responses to both Return and Callback URLs, which confirms that the response really did come from us. Here are the steps to calculate the response hash value:
-
First, sort response parameters - excluding hash - alphabetically and then concatenate. Here is an example:
amount . cardnumber . code . message . merchant_order_id . transaction_id //14.99XXXXXXXXXXXX87101Transaction Success201706191113454353
-
SHA256 encrypt the resulting string:
15dc8221a54a05e9c72f360a4468ccbc26946ad931e95e48f27e5845c79a7563
-
Append your company hash to the string generated in step two, and SHA256 encrypt again::
15dc8221a54a05e9c72f360a4468ccbc26946ad931e95e48f27e5845c79a7563company_hashcode
b1d09abc0be3a5beb86aa5b9b6b70e1fff26f68eb97ba7f8f0be4d506396b2dd
Your company_hashcode value is waiting for you, just ping the support@acquired.com team.
All done? Here are samples of code to calculate the response hash value:
$response = array( 'transaction_id'=>212327, 'company_id'=>113, 'merchant_order_id'=>'20160709_666', 'company_mid_id'=>'1025', 'currency_code_iso3'=>'GBP', 'code'=>1, 'amount'=>100.00, 'message'=>'Declined', 'billing_country_code_iso2'=>'GB', 'billing_city' => 'London', 'billing_state' => 'London', 'billing_street' => '123 Fake Street', 'billing_street2' => 'Hammersmith', 'billing_zipcode'=>'W120LU', 'billing_email'=>'test@sample.com', 'shipping_country_code_iso2'=>'GB', 'shipping_city'=>'London', 'shipping_state'=>'London', 'billing_zipcode'=>'W120LU', 'shipping_street'=>'', 'shipping_street2'=>'', 'shipping_zipcode'=>'', 'shipping_email'=>'test@sample.com' ); $company_hashcode = 'hashcode'; ksort($response); //100LondonGBtest@sample.comLondon123 Fake StreetHammersmithW120LU11131025GBP20160709_666DeclinedLondonGBtest@sample.comLondon212327 //Hash before add company hash code: 72ad0f53cf1e0c3102ebff9edd2b178e8fd4f87cdc468f0267ddb6afe3b28876 $response['hash'] = hash('sha256', hash('sha256', implode('', $response)).$company_hashcode); //Hash: 7c28394b9bb5631c5d8916a16cab2c0b5a59bd7a583f71ef9baa706f0bf1f676
private String getHppHash() { String companyHash="hashcode"; String hppHash=""; Map
map = new HashMap (); map.put("amount", 100.00); map.put("transaction_id", 212327 ); map.put("amount", 100.00 ); map.put( "company_id", 113 ); map.put( "company_mid_id", 1025 ); map.put( "currency_code_iso3", "GBP" ); map.put( "code", 1 ); map.put( "merchant_order_id", "20160709_666" ); map.put( "message", "Declined" ); map.put( "billing_country_code_iso2", "GB" ); map.put( "billing_city", "London" ); map.put( "billing_state", "London"); map.put( "billing_street", "123 Fake Street"); map.put( "billing_street2", "Hammersmith"); map.put( "billing_zipcode", "W120LU"); map.put( "billing_email", "test@sample.com"); map.put( "shipping_country_code_iso2", "GB"); map.put( "shipping_city", "London"); map.put( "shipping_state", "London"); map.put( "shipping_street", ""); map.put( "shipping_street2", ""); map.put( "shipping_zipcode", ""); map.put( "shipping_email", "test@sample.com"); Map sortMap = new TreeMap (new MapKeyComparator()); sortMap.putAll(map); StringBuffer sb = new StringBuffer(); for (Iterator iterator = sortMap.entrySet().iterator(); iterator.hasNext(); ) { Map.Entry entry = (java.util.Map.Entry) iterator.next(); sb.append(null == entry.getValue() ? "" : entry.getValue().toString()); } String plain = sb.toString(); // plain = "100LondonGBtest@sample.comLondon123 Fake StreetHammersmithW120LU11131025GBP20160709_666DeclinedLondonGBtest@sample.comLondon212327"; String temp = String2SHA256(sb.toString()); // Hash before add company hash code: 72ad0f53cf1e0c3102ebff9edd2b178e8fd4f87cdc468f0267ddb6afe3b28876 hppHash = String2SHA256( temp + companyHash); // Hash: 7c28394b9bb5631c5d8916a16cab2c0b5a59bd7a583f71ef9baa706f0bf1f676 } private static String String2SHA256(String str) { MessageDigest messageDigest; String encodeStr = ""; try { messageDigest = MessageDigest.getInstance("SHA-256"); messageDigest.update(str.getBytes("UTF-8")); encodeStr = byte2Hex(messageDigest.digest()); } catch (NoSuchAlgorithmException e) { e.printStackTrace(); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } return encodeStr; } private static String byte2Hex(byte[] bytes) { StringBuffer stringBuffer = new StringBuffer(); String temp = null; for (int i = 0; i < bytes.length; i++) { temp = Integer.toHexString(bytes[i] & 0xFF); if (temp.length() == 1) { stringBuffer.append("0"); } stringBuffer.append(temp); } return stringBuffer.toString(); } class MapKeyComparator implements Comparator { @Override public int compare(String str1, String str2) { return str1.compareTo(str2); } } private string getResponseHash() { string companyHashCode = "hashcode"; Dictionary
param = new Dictionary { { "transaction_id", 212327 }, { "amount", 100.00 }, { "company_id", 113 }, { "company_mid_id", 1025 }, { "currency_code_iso3", "GBP" }, { "code", 1 }, { "merchant_order_id", "20160709_666" }, { "message", "Declined" }, { "billing_country_code_iso2", "GB" }, { "billing_city", "London" }, { "billing_state", "London" }, { "billing_street", "123 Fake Street" }, { "billing_street2", "Hammersmith" }, { "billing_zipcode", "W120LU" }, { "billing_email", "test@sample.com" }, { "shipping_country_code_iso2", "GB" }, { "shipping_city", "London" }, { "shipping_state", "London" }, { "shipping_street", "" }, { "shipping_street2", "" }, { "shipping_zipcode", "" }, { "shipping_email", "test@sample.com" } }; Dictionary sorted = param.OrderBy(o => o.Key).ToDictionary(o => o.Key, p => p.Value); string plain = string.Empty; foreach (KeyValuePair k in sorted) { plain += k.Value; } ViewData["ResponsePlain"] = plain; //1.Plain string:100LondonGBtest@sample.comLondon123 Fake StreetHammersmithW120LU11131025GBP20160709_666DeclinedLondonGBtest@sample.comLondon212327 string temp = SHA256(plain); //2.Hash before add company hash code: 72ad0f53cf1e0c3102ebff9edd2b178e8fd4f87cdc468f0267ddb6afe3b28876 ViewData["ResponseBeforeCompanyHash"] = temp; string hppHash = SHA256(temp + companyHashCode); //3.Hash: 7c28394b9bb5631c5d8916a16cab2c0b5a59bd7a583f71ef9baa706f0bf1f676 return hppHash; } private static string SHA256(string str) { byte[] SHA256Data = Encoding.UTF8.GetBytes(str); SHA256Managed Sha256 = new SHA256Managed(); byte[] by = Sha256.ComputeHash(SHA256Data); return BitConverter.ToString(by).Replace("-", "").ToLower(); //64 }
Response Parameters
Parameter | Format | Length | Description |
---|---|---|---|
amount | int |
1-11 | Transaction amount. |
authorization_code | string |
0-50 | Authorisation code returned by the issuing bank. |
avsaddress | string |
1-2 | AVS check on the first line of the customer’s address, when available. |
avszipcode | string
| 1-2 | AVS check on the first line of the customer’s postcode or zipcode, when available. |
bank_response_code | int |
2 | Transaction result code communicated by the issuing bank. |
bin | int |
6 | First six characters of the card used for the transaction. |
card_category | string |
0-30 | Category of the card used, for example CREDIT or DEBIT. |
card_level | string |
0-30 | Level of the card used, for example CLASSIC. |
card_type | string |
2-8 | Type of card used for the transaction, possible values are VISA, MC, AMEX. |
cardexp | int |
6 | Expiration date of the card used for the transaction, formatted as MMYYYY. |
cardholder_name | string |
2-45 | Cardholder’s name as entered into the HPP. |
cardnumber | string |
12-19 | Masked credit or debit card number used for the transaction. |
code | int |
1-3 | Code describing the transaction results. |
company_id | int |
1-4 | API Company ID we issue to merchants. |
company_mid_id | int |
1-11 | API MID ID we issue to merchants. |
currency_code_iso3 | string |
3 | Transaction currency, an ISO 4217 3-digit code. |
eci 3-D Secure |
int |
1-2 | Result of 3-D Secure authentication. |
cvvresult | string |
1-2 | Result of CVV2 security check. |
is_remember | boolean |
1 | Flag to indicate if we have tokenised card details for future use. |
issuing_bank | string |
0-50 | Name of the bank issuing the card used for the transaction. |
issuing_country | string |
0-50 | Country that issued the card used for the transaction. |
issuing_country_iso2 | string |
0-2 | Country that issued the card used for the transaction, an ISO2 two-letter code. |
merchant_order_id | string |
0-50 | Unique ID you’ll have used to identify each transaction, repeated from the request. |
message | string |
1-65535 | Text describing the transaction response. |
transaction_id | int |
1-10 | Unique ID we generate to identify the transaction. |
hash | string |
10-2083 | Verification hash value. See here. |
Styling
This is the fun part - it’s time to customise your payment page. We’ve added a default template to the Hub to get you started. Download and change it however you want (within reason), please take a look below for further information on how to download the default template.
Your customers will have a better checkout experience, and by hosting your CSS, JavaScript, image and font files, we take on the burden of costly and complex PCI DSS compliance. You just need to complete SAQ A. How cool is that?
To make our solution as flexible as acceptable, we assign a placeholder to each element of the payment form. These simply display information passed through the request or gather additional information during checkout.
Uploading the Template
Templates can be uploaded in the Settings section of our portal. To upload a template, go to Settings > MIDs and from the Action dropdown select Create / Edit Service. Not sure if you’re in the right place? Take a look at the screenshot below:
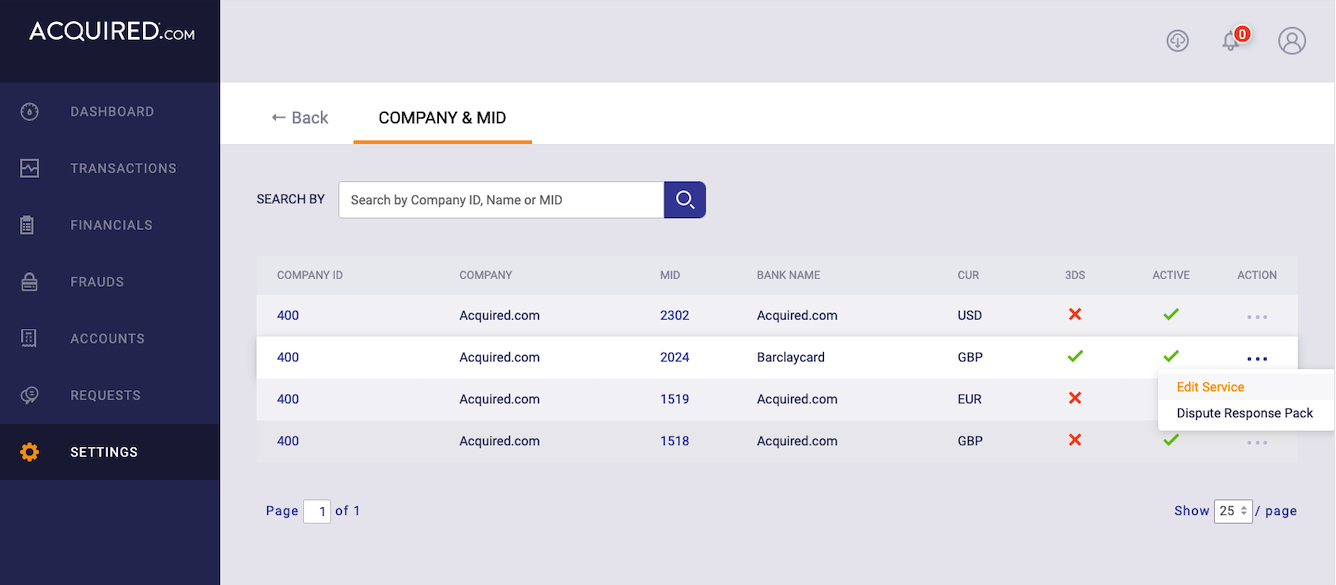
You must name the HTML file index.html. All files must be in one zipped file without any additional folders.
Default Template
You can set a default template in the Hub which displays if the template_id
parameter isn’t populated in the request. Here’s a handy screen shot:
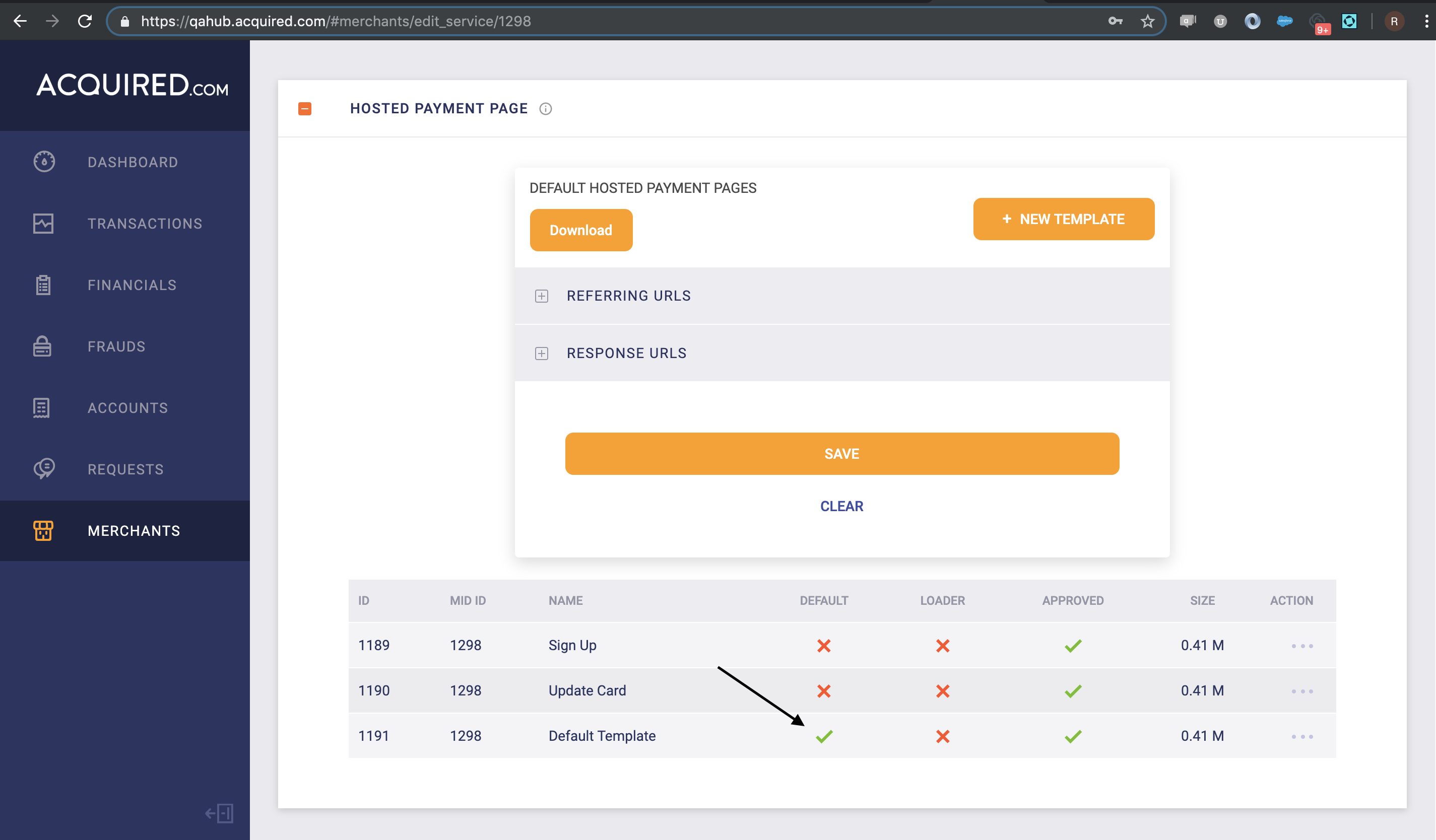
Dynamic Template
To change the template displayed to customers dynamically, you must include the template_id
parameter in the HPP request and assign a unique identifier to each uploaded file. This value displays in the HPP section of the Hub once your template is created. Sounds complicated, but it’s really not:
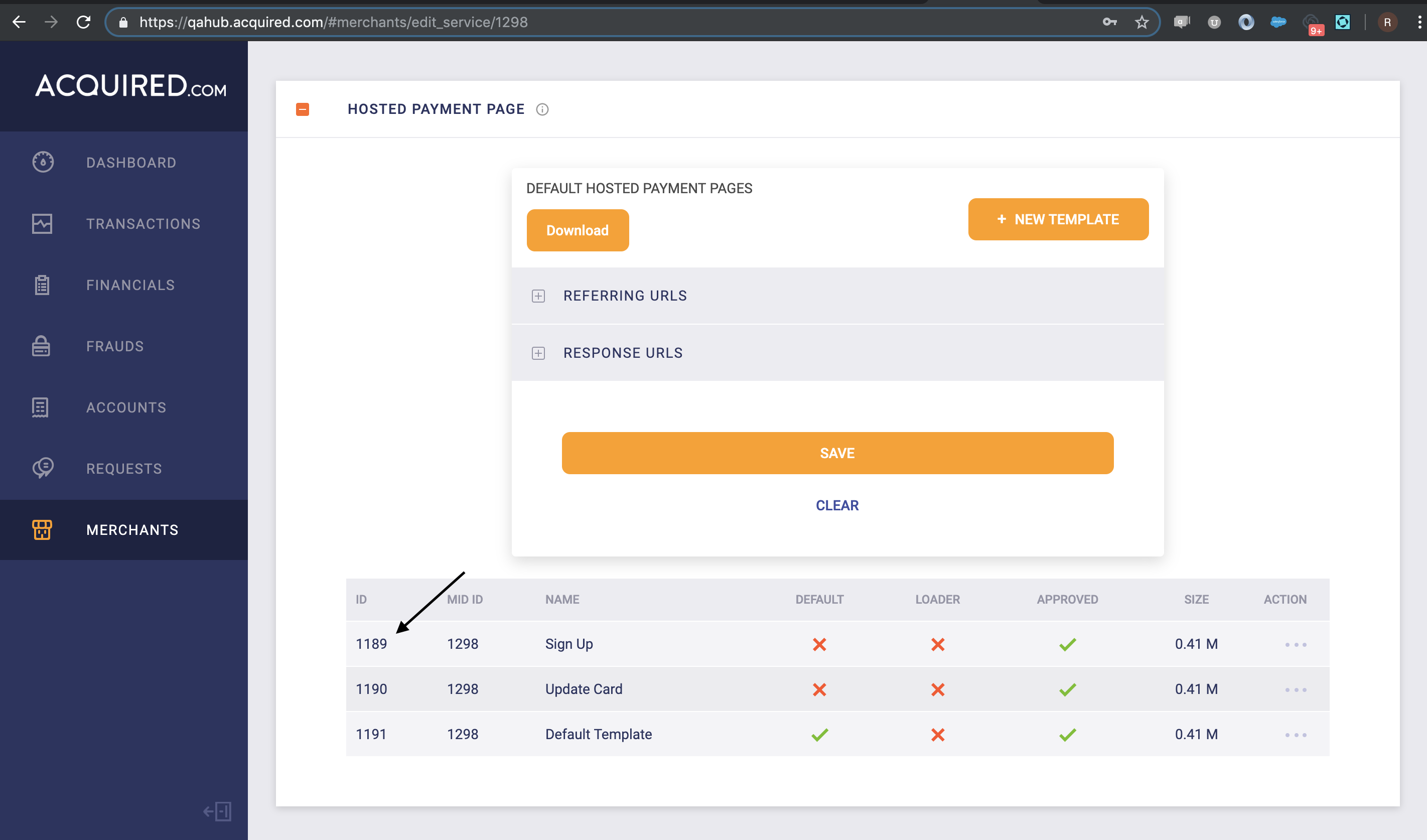
Placeholders - Card Details
When you create the all-important payment form, use the following placeholders as values in the HTML:
Parameter | Placeholder | Description |
---|---|---|
cardholder_name | {{cardholder_name}} | Cardholder name. |
cardnumber | {{cardnumber}} | Card number. |
cardexp | {{cardexp_month}} | Card expiry month. |
cardexp | {{cardexp_year}} | Card expiry year. |
cardcvv | {{cardcvv}} | CVV2 value. |
subscription_type | {{is_remember}} | Flag to initiate a INIT subscription. |
Want to know how to incorporate these placeholders into your template? There’s an example in the portal. For a test account, contact support@acquired.com.
Payment Links
With a small change to the endpoint - instead of immediately redirecting your customers to the Hosted Payment Page (HPP), we will return a Payment Link.
This link could be embedded in an email, sent as a text message or just used to load the payment form on your website.
Endpoints
Just add /link to the HPP endpoint and you are good to go.
Security
Request Hash
The hash
value calculation is the same - take a look here.
Request
The request parameters are identical with two additions:
Parameter | Format | Length | Description |
---|---|---|---|
expiry_time |
string |
1-7 | The time in seconds after which you would like the link to expire. By default it is set to one weeks but you can set this value up to a 12 weeks, afterwhich we will expire the link on our end. For example, 24 hours = 86400. Acceptable Characters: 0-9 |
retry_attempts |
string |
1-2 | This is the number of times you would like a user to be able to retry a payment using the link provided before the link becomes inactive and will no longer be able to be used. By default it is set to 3 but you can set this value up to a 10 attempts Acceptable Characters: 0-9 |
Request Message
Thankfully, since there is no immediate redirect, you can submit the request in JSON format like below:
Response
What you will get back is a Payment Link URL to use as you wish: